Http Retry Support
- Date
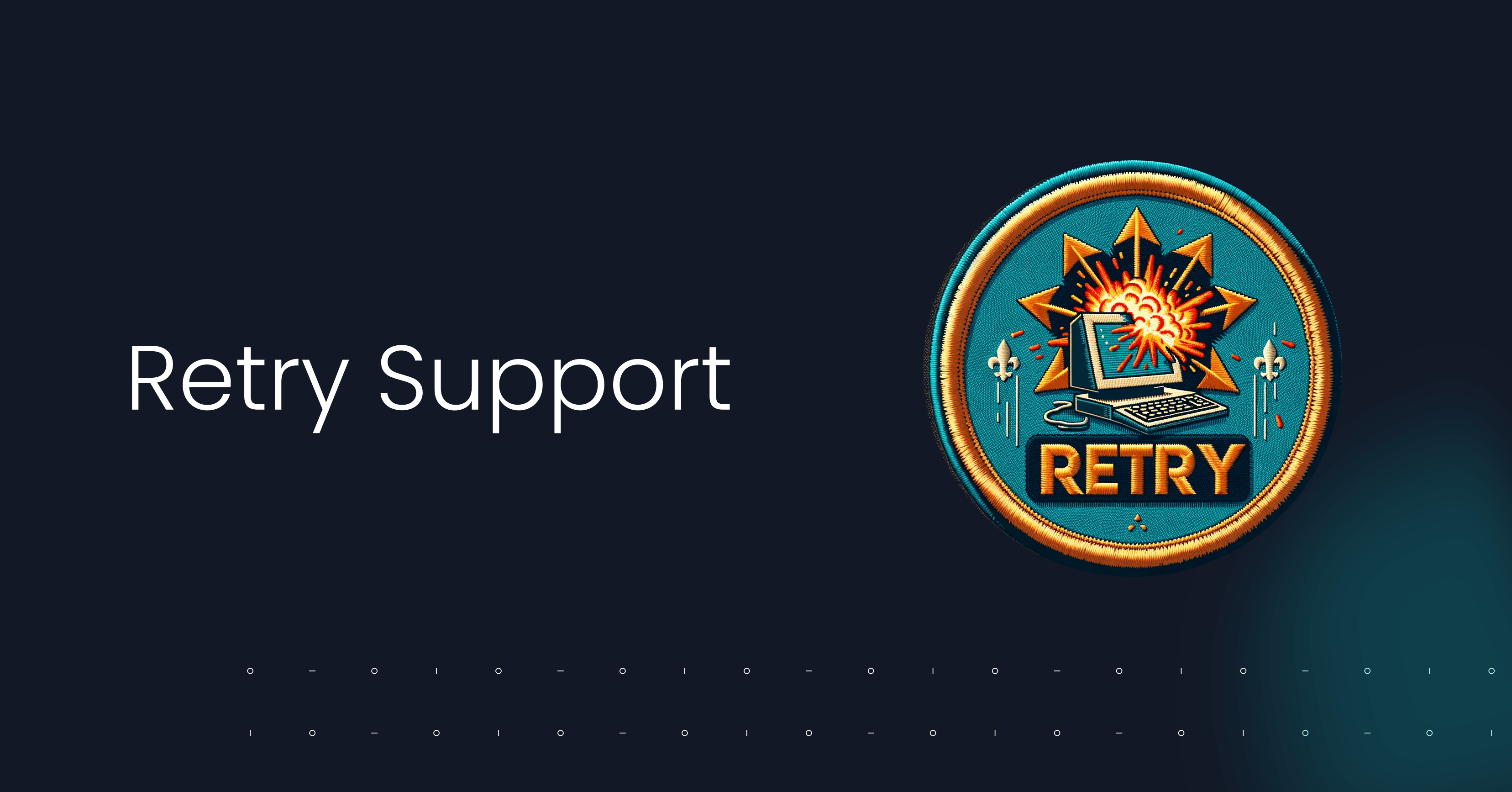
Retry Option for Http operations:
Let’s say you need to stitch Film data with reviews coming from an unreliable third party Rest Api:
service ReviewsApi {
@HttpOperation(method = "GET", url = "https://reviews/{id}")
operation getReviews(id: FilmId): FilmReview[]
}
The third party REST API gives you intermittent HTTP 500s as it can’t cope with user requests at particular times during day.
To address such outages, you can now define a HttpRetry
settings in Taxi for the operation.
Orbital Supports two HttpRetry
policies:
Retry Policy with Fixed Delay
Let say you want to Orbital to retry ‘getReviews’ operation when it responds with Http 500. You want Orbital to try the operation at most 10 times and wait 5 seconds between each retry. Here is how you can modify the taxi definition:
service ReviewsApi {
@HttpOperation(method = "GET", url = "https://reviews/{id}")
@HttpRetry(responseCode = [500], fixedRetryPolicy = @HttpFixedRetryPolicy(maxRetries = 10, retryDelay = 5))
operation getReviews(id: FilmId): FilmReview[]
}
You can trigger the retry mechanism for multiple response codes as well, here is how you can modify previous Taxi definition to trigger the Retry mechanism when the remote service returns either Http 500 or Http 502
service ReviewsApi {
@HttpOperation(method = "GET", url = "https://reviews/{id}")
@HttpRetry(responseCode = [500, 502],
fixedRetryPolicy = @HttpFixedRetryPolicy(
maxRetries = 10,
retryDelay = 5
))
operation getReviews(id: FilmId): FilmReview[]
}
Retry Policy with Exponential Delay
With exponential delay retry policy, Orbital will wait progressively longer intervals between consecutive retries:
service ReviewsApi {
@HttpOperation(method = "GET", url = "https://reviews/{id}")
@HttpRetry(responseCode = [500, 502], fixedRetryPolicy = @HttpExponentialRetryPolicy(maxRetries = 10, retryDelay = 5, jitter = 0.5))
operation getReviews(id: FilmId): FilmReview[]
}
With the above definition, Orbital will try invoking the Rest at most 10 times in case of Http 500 or Http 502 errors.
However, the delay between retries will increase exponentially.
With a jitter
value between 0 and 1, you add some randomness to the delay time by introducing a random delay, or “jitter”, to the next retry delay time.
This ensures that the retries are not synchronous and reduces the likelihood of a retry storm.
Here’s how the table would look with exponential backoff with Jitter:
Retry Attempt | Delay Time (seconds) | Jitter Range (seconds) | Actual Delay Time (seconds) |
---|---|---|---|
1 | 1.0 | 0.5 | 1-0 - 1.5 |
2 | 2.0 | 0.5 | 1-5 - 2.5 |
3 | 4.0 | 0.5 | 3.5 – 4.5 |
4 | 8.0 | 0.5 | 7.5 – 8.5 |
5 | 16.0 | 0.5 | 15.5 – 16.5 |
Summary
Http retries is out in 0.27. Read more about it over in our docs