Orbital 0.35 - Partial models, mixed-source projects, parallel streaming, improved caching
- Date
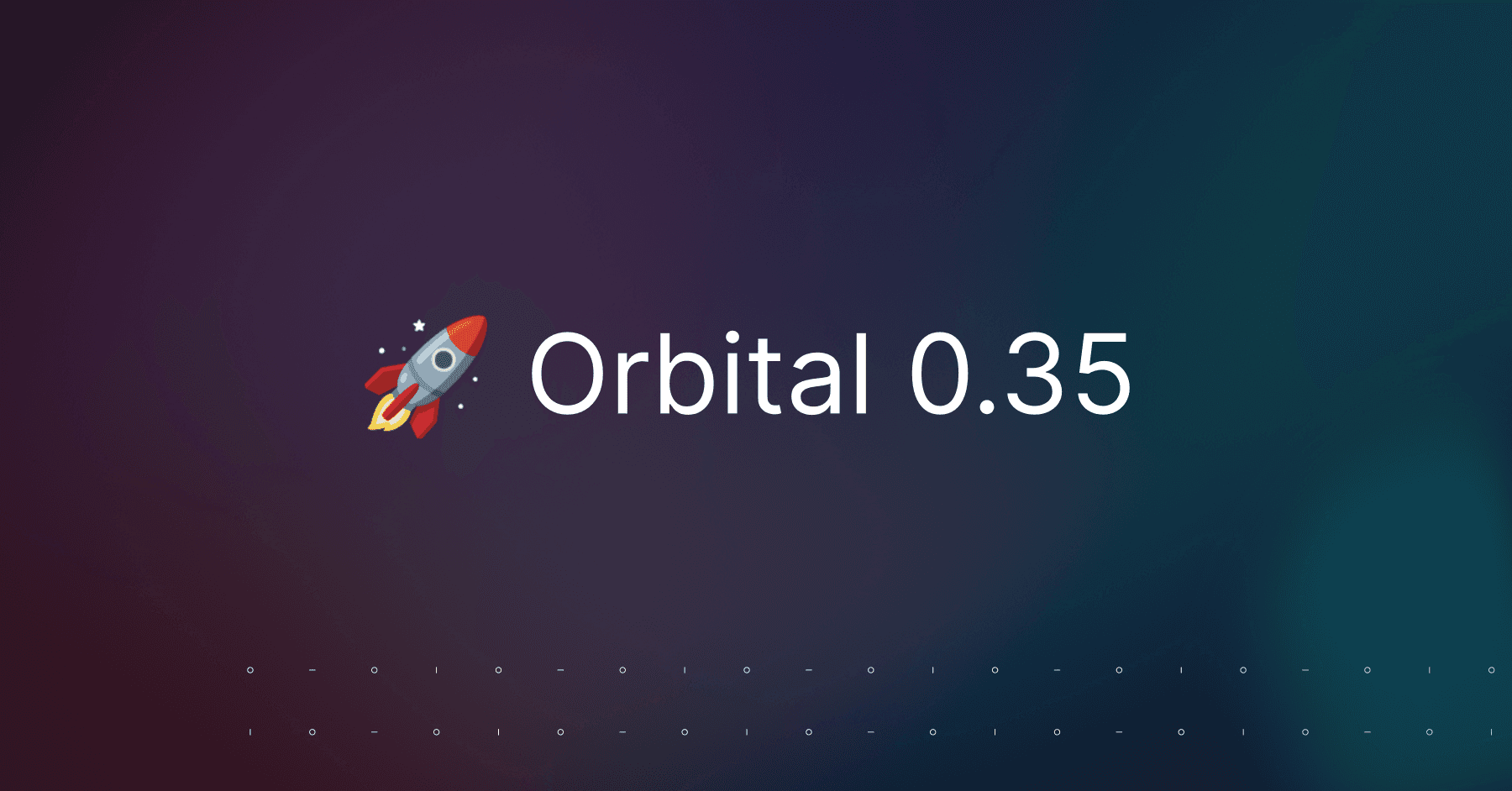
Orbital 0.35.0 brings significant enhancements to core capabilities, with major improvements to streaming, caching, and model handling.
This release focuses on stability and performance, particularly for high-volume data processing scenarios.
Partial models
Partial models create variations of existing models where all fields are optional. This pattern is especially useful for API operations that support partial updates (PATCH), allowing clients to send only the fields they want to change.
model Customer {
id: CustomerId inherits String
name: CustomerName inherits String
}
// Creates a new model where all fields are optional
partial model CustomerUpdate from Customer
When you create a partial model, all fields become optional (nullable), including:
- Nested objects: Automatically converted to partial versions
- Collections: Arrays become arrays of partial versions
- Self-references: Updated to reference the partial version
- Annotations from the source model are merged with annotations on the partial model
- Modifiers are inherited with one exception: partial models cannot be
closed
This all combines well with the @OmitNulls annotation to ensure null fields are excluded from serialized payloads:
// Original model with required fields
model Product {
id: ProductId inherits String
name: ProductName inherits String
price: Price inherits Decimal
category: Category
tags: Tag[]
}
// Create a partial version where everything is optional
@OmitNulls
partial model ProductUpdate from Product
service ProductCatalog {
// Full replacement (PUT)
write operation replaceProduct(Product): Product
// Partial update (PATCH)
write operation updateProduct(ProductUpdate): Product
}
OpenAPI, Avro and Protobuf in a taxi project
We now support including OpenAPI, Avro and Protobuf files directly within a taxi project.
Add entries in the additionalSources
block of your taxi.conf
, as shown below:
name: taxi/mixed-sources
version: 0.3.0
sourceRoot: src/
additionalSources: {
"@orbital/avro" = "avro/src/*.avsc"
"@orbital/openapi" = "openapi/*.yaml"
"@orbital/protobuf" = "proto/src/**/*.proto"
}
These sources are automatically read, along with their taxi annotations, and imported into your taxi project.
This simplifies setups (and startup times) for teams where each spec was previously imported as standalone projects.
Parallel streaming
When running Orbital in a cluster, background stream jobs can now be configured to run in parallel, which controls the number of nodes in the cluster that will execute the stream.
using the @Parallel
annotation.
For example, assuming Orbital is deployed in a 6-node cluster, consuming events from Kafka.
import com.orbitalhq.streams.Parallel
@Parallel(count = 4)
query MyStream {
stream { CustomerLoggedInEvent }
call MyMongoDb::upsertCustomerMetrics
}
In this configuration, 4 of the nodes will subscribe to Kafka, and consume the events.
Orbital correctly rebalances as nodes enter and leave the cluster.
Learn more in the streaming data docs
Improved caching controls
The @com.orbitalhq.caching.Cache
annotation offers control over caching behavior at both operation and model
levels.
You can disable caching for specific operations or data types, or adjust cache expiration with configurable time-to-live settings.
This helps balance performance needs when working with both static reference data and changing values in long-running stream processors.
Read more about configuring caching in the caching docs
Azure service bus
We added support for Azure Service Bus, enabling integration with Azure messaging infrastructure through a full-featured connector.
Read more in the Azure Service Bus docs
Other updates
Enhanced Model & Query Capabilities
- Nested Projections with Multiple Scopes: Fixed support for nested projections with multiple scope variables, eliminating the “How to handle multiple scoped facts here?” error.
- Operation Input Improvements: Operations can now accept inputs populated by supertypes, enabling more flexible service invocation.
- Added ability to use expressions and constraints on the left-hand side of type references. [ORB-932]
- Object build type searches now correctly consider constraints. [ORB-895]
- Improved support for constraints on nested chained projections. [ORB-897]
- Spread Operator Improvements: Fixed spread operator to properly consider inherited types. [ORB-891]
Streaming Enhancements
- Added low-cardinality error reporting for streams, providing better visibility into failures during stream processing.
- Fixed error reporting in streams so stream failures are accurately reported in the UI. [ORB-868]
- Streaming queries with a mutation operation now continue even if the mutation operation fails. [ORB-934]
HTTP Improvements
- HTTP Retry Reporting: Fixed reporting of retried HTTP service calls in the profile window, improving visibility of retry attempts for operations with the
@HttpRetry
annotation. [ORB-931]
Operational Improvements
- Improved Error Reporting: Enhanced error reporting when variables are missing in queries, providing more meaningful feedback to developers.
- Metrics & Monitoring: Multiple improvements to stream observability, including better error reporting, status monitoring, and metrics collection.
File Monitoring
- Git Project File Watching: Added support for polling file watchers with Git projects, providing more reliable file change detection in certain environments. [ORB-865]
- Empty Workspace Configuration: Added ability to configure file monitoring settings for automatically created empty workspaces.
- Authentication Improvements: Fixed issue where updates in auth.conf were not reflected in Orbital Query Execution. [ORB-943]