Guides
Working with XML and JSON
This guide showcases services publishing a mix of XML and JSON, how to combine them, and expose the composed services as a REST API.
It shows starting an Orbital project from scratch, so if you’re unfamiliar with Orbital, this is the perfect starting point.
This demo has a video walkthrough, talking about how it’s built:
Overview
This guide shows connecting:
// A service the returns a list of Films (in XML)
operation findAllFilms():FilmList
// A service that returns the cast of a film (in XML)
operation findCast(FilmId):ActorList
// A service that returns the list of awards a film has won (in JSON)
operation findAwards(FilmId):Award[]
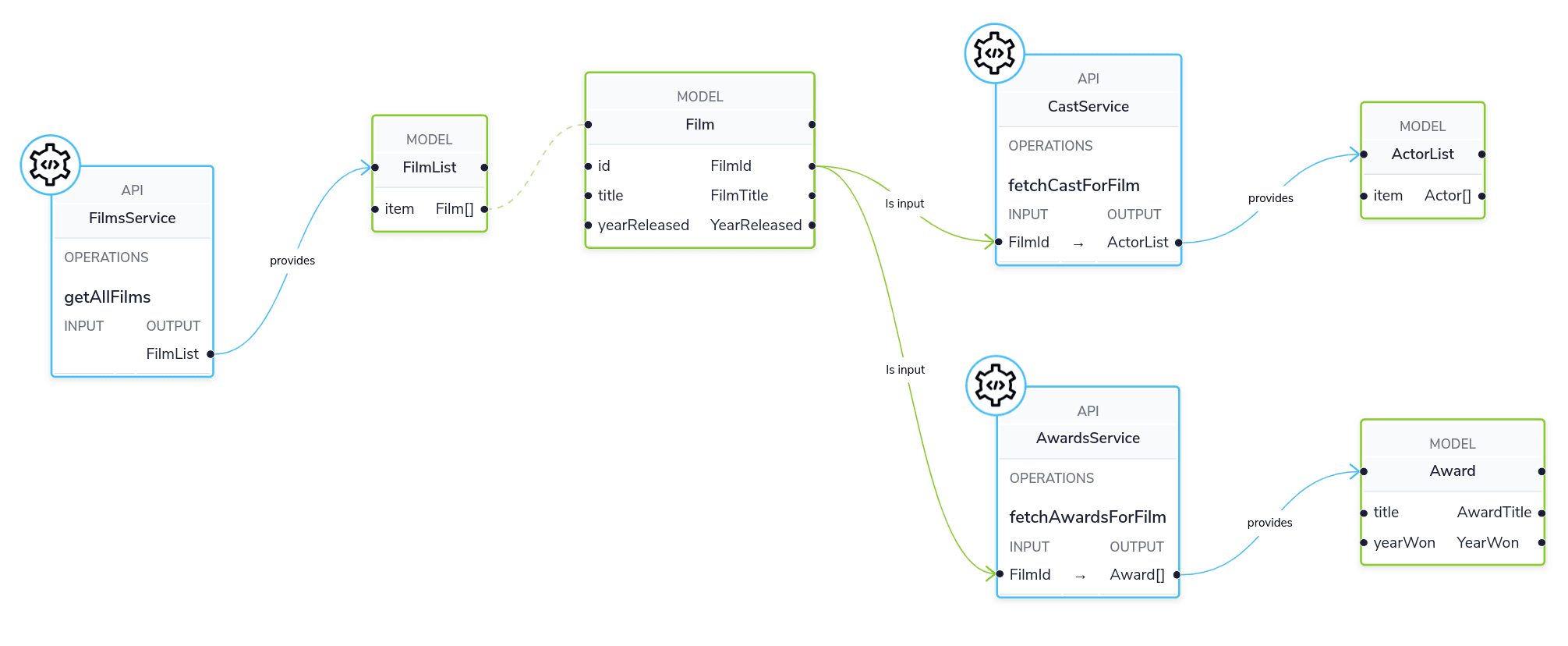
We’ll stitch these together, and expose a REST API that returns the data from all 3 services.
Setting Up
Jump to this section of the video
To get started, install Taxi using SDKMan, and create a new project:
sdk install taxi
taxi init
# follow prompts to create your taxi project
Then launch an Orbital developer environment.
taxi orbital
This downloads a docker-compose file which configures a local developer environment of Orbital, which live-reloads your Taxi project changes.
Describing the Film Service
Jump to this section of the video
Write some Taxi that describes our Film service.
import com.orbitalhq.formats.Xml
// The @Xml annotation tells Orbital how to read this object
@Xml
model FilmList {
item : Film[]
}
model Film {
id : FilmId inherits Int
title : FilmTitle inherits String
yearReleased : YearReleased inherits Int
}
service FilmsService {
@HttpOperation(url = "http://localhost:8044/films", method = "GET")
operation getAllFilms():FilmList
}
<List>
<item id="0">
<title>ACADEMY DINOSAUR</title>
<yearReleased>2005</yearReleased>
</item>
<item id="1">
<title>ACE GOLDFINGER</title>
<yearReleased>1975</yearReleased>
</item>
</List>
Integrating the Cast Service
Our CastService takes a the Id of a Film, and returns a list of Actors:
import com.orbitalhq.formats.Xml
@Xml
model ActorList {
item : Actor[]
}
model Actor {
id : ActorId inherits Int
name : ActorName inherits String
}
service CastService {
@HttpOperation(url = "http://localhost:8044/film/{filmId}/cast", method = "GET")
operation fetchCastForFilm(@PathVariable("filmId") filmId : FilmId):ActorList
}
<List>
<item>
<id>34</id>
<name>JUDY DEAN</name>
</item>
<item>
<id>21</id>
<name>ELVIS MARX</name>
</item>
</List>
What connects it together
Orbital now has enough information to link our services together:
// The FilmId from our Film model...
model Film {
id : FilmId inherits Int
...
}
// ... is used as an input to our fetchCastForFilm operation:
operation fetchCastForFilm(FilmId):ActorList
We don’t need to write any integration code, or resolvers. There’s enough information in the schemas.
Reminder
If these services published XSDs or WSDLs, we could’ve leveraged them, and only needed to declare the Taxi scalars, such as FilmId
Writing Data Queries
Jump to this section of the video
Orbital uses type metadata to understand how to link things together. Rather than writing integration code, we write a query for data using TaxiQL.
Fetch the list of films
// Just fetch the ActorList
find { FilmList }
Which returns:
{
"item": [
{
"id": 0,
"title": "ACADEMY DINOSAUR",
"yearReleased": 2005
},
{
"id": 1,
"title": "ACE GOLDFINGER",
"yearReleased": 1975
},
// snip
]
}
Restructure the result
We’d like to remove the item
wrapper (which is carried over from the XML format), so we change the query, to ask just for a Film[]
find { FilmList } as Film[]
Which returns:
[
{
"id": 0,
"title": "ACADEMY DINOSAUR",
"yearReleased": 2005
},
{
"id": 1,
"title": "ACE GOLDFINGER",
"yearReleased": 1975
}
]
Defining a custom response object
We can define a data contract of the exact data we want back, specifying the field names we like, with the data type indicating where the data is sourced from:
find { FilmList } as (Film[]) -> {
filmId : FilmId
nameOfFilm : FilmTitle
}
Linking our actor service
To include data from our CastService
, we just ask for the actor information:
find { FilmList } as (Film[]) -> {
filmId : FilmId
nameOfFilm : FilmTitle
cast : Actor[]
}
Which now gives us:
{
"filmId": 0,
"nameOfFilm": "ACADEMY DINOSAUR",
"cast": [
{
"id": 18,
"name": "BOB FAWCETT"
},
{
"id": 28,
"name": "ALEC WAYNE"
},
//..snip
]
}
Adding our Awards service
We can also define a schema and service for our Awards information, which is returned in JSON:
model Award {
title : AwardTitle inherits String
yearWon : YearWon inherits Int
}
service AwardsService {
@HttpOperation(url = "http://localhost:8044/film/{filmId}/awards", method = "GET")
operation fetchAwardsForFilm(@PathVariable("filmId") filmId : FilmId):Award[]
}
[
{
"title": "Best Makeup and Hairstyling",
"yearWon": 2020
},
{
"title": "Best Original Score",
"yearWon": 2020
},
// snip...
]
Enriching our query
Finally, to include this awards data, we just add it to our query:
find { FilmList } as (Film[]) -> {
filmId : FilmId
nameOfFilm : FilmTitle
cast : Actor[]
awards : Award[]
}
Which gives us:
{
"filmId": 0,
"nameOfFilm": "ACADEMY DINOSAUR",
"cast" : [] // omitted
"awards": [
{
"title": "Best Documentary Feature",
"yearWon": 2020
},
{
"title": "Best Supporting Actress",
"yearWon": 2020
},
]
}
Publishing our Query as REST API
Now that we’re happy with our response data, we can publish this query as a REST API.
- First, we wrap the query in a
query { ... }
block, and save it in our taxi project - Then we add an
@HttpOperation(...)
annotation.
@HttpOperation(url = '/api/q/filmsAndAwards', method = 'GET')
query filmsAndAwards {
find { FilmList } as (Film[]) -> {
filmId : FilmId
nameOfFilm : FilmTitle
awards : Award[]
cast : Actor[]
}[]
}
Our query is now available at http://localhost:9022/api/q/filmsAndAwards
$ curl http://localhost:9022/api/q/filmsAndAwards | jq
Gives us:
[
{
"filmId": 0,
"nameOfFilm": "ACADEMY DINOSAUR",
"awards": [
{
"title": "Best Animated Feature",
"yearWon": 2020
},
{
"title": "Best Original Score for a Comedy",
"yearWon": 2020
},
{
"title": "Best Documentary Feature",
"yearWon": 2020
},
// .... snip
]
}
]
Wrapping Up and Next Steps
Throughout this guide, we’ve:
Created a Taxi project
Exposed XML services, and modelled their responses
Written a query stitching three services together
Published that query as an HTTP service
The code for this guide is available on Github.
Remember, if you haven’t done so, head to the Orbital github repo, and give us a Star!